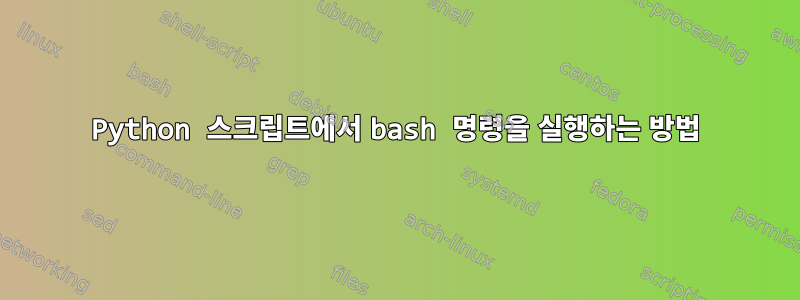
sudo apt update
Python 스크립트에서 여러 인수(예: "")를 사용하여 bash 명령을 시작하는 방법은 무엇입니까 ?
답변1
@milne의 답변은 효과가 있지만 subprocess.call()
피드백은 거의 제공되지 않습니다.
subprocess.check_output()
나는 stdout에 인쇄된 내용을 분석할 수 있도록 다음 을 사용하는 것을 선호합니다 .
import subprocess
res = subprocess.check_output(["sudo", "apt", "update"])
for line in res.splitlines():
# process the output line by line
check_output
명령 호출이 0으로 종료되면 오류가 발생합니다.
bash
함수에 키워드 인수를 지정하지 않으면 이는 호출이나 다른 셸을 호출하지 않습니다 (Call the command shell
의 경우에도 마찬가지입니다subprocess.call()
Python에서 (다른) 명령 호출을 많이 하는 경우 다음을 살펴보는 것이 좋습니다.선두. 이렇게 하면 (IMO) 더 읽기 쉽게 됩니다.
from plumbum.cmd import sudo, apt, echo, cut
res = sudo[apt["update"]]()
chain = echo["hello"] | cut["-c", "2-"]
chain()
답변2
bash를 프로그램으로 사용하고 -c 매개변수를 사용하여 명령을 실행할 수 있습니다.
예:
bashCommand = "sudo apt update"
output = subprocess.check_output(['bash','-c', bashCommand])
답변3
이것하위 프로세스모듈은 다음을 수행하도록 설계되었습니다.
import subprocess
subprocess.call(["sudo", "apt", "update"])
명령이 실패할 때 스크립트를 종료하려면 check_call()
반환 코드를 직접 구문 분석하는 대신 다음을 사용하는 것이 좋습니다.
subprocess.check_call(["sudo", "apt", "update"])
답변4
Python 3.5 이상의 경우 다음을 사용할 수 있습니다.
import subprocess
try:
result = subprocess.run("sudo apt update", check=True, shell=True, stdout=subprocess.PIPE, stderr=subprocess.PIPE)
except subprocess.CalledProcessError as err:
raise Exception(str(err.stderr.decode("utf-8")))
except Exception as err:
raise Exception(err)
else:
return result.stdout.decode("utf-8")