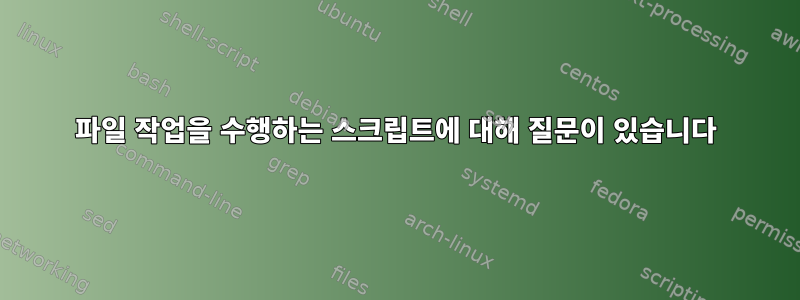
저는 다운로드 디렉터리에 생성된 파일을 즉시 감지한 다음 파일 확장자에 따라 정렬하는 스크립트를 개발해 왔습니다. 그러나 문제는 인터넷에서 파일을 다운로드할 때 파일이 이동되고 다운로드로 인해 파일이 손상될 수 있다는 것입니다.
완료될 때까지 스크립트 작업을 중지하는 방법이 있습니까? 예를 들어 인터넷에서 파일을 다운로드할 때 일반적으로 .part 파일 확장자를 가진 파일이 표시되고 무언가를 추가할 수 있으며 이 파일 확장자가 감지되면 사라질 때까지 반복할까요?라는 아이디어가 있었습니다. 그런 다음 나머지 스크립트를 계속 실행하시겠습니까?
어떤 제안이라도 받아들이고 싶지 않다면 질문이 있으면 물어보세요. -감사해요
#!/bin/bash
# Requires inotify-tools package
#
# Authors: oddstap && yetanothergeek
#
# This simple tool takes newly created files in the Downloads directory
# and then organizes them based on file extension.
TARGET=$HOME/Downloads
inotifywait -m -e close_write -e moved_to --format "%f" "$TARGET" \
| while read FILENAME; do
EXT=${FILENAME##*.} # Extract file extension
EXT=${EXT,,} # Convert to lowercase
DEST_DIR=''
case "$EXT" in
# Word processor and text files
doc|docx|odt|pdf|rtf|tex|txt|wks|wps|wpd)
DEST_DIR="$HOME/Documents/Word_Processor_And_Text_files"
;;
# Audio files
mp3|wav|wma|mid|midi|aif|cda|mpa|ogg|wpl)
DEST_DIR="$HOME/Music"
;;
# Image files
jpg|jpeg|png|ai|bmp|gif|ico|ps|svg|tif|tiff|psd)
DEST_DIR="$HOME/Pictures"
;;
# Video files
avi|wmv|3g2|3gp|flv|h264|m4v|mkv|mov|mp4|mpg|mpeg|rm|swf|vob|wmv)
DEST_DIR="$HOME/Videos"
;;
# Compressed files
7z|arj|deb|pkg|rar|rpm|gz|z|zip)
DEST_DIR="$HOME/Documents/Compressed_Files"
;;
# Disc and media files
bin|dmg|iso|toast|vcd)
DEST_DIR="$HOME/Documents/Disk_Images"
;;
# Data and database files
csv|dat|db|dbf|log|mdb|sav|sql|tar|xml)
DEST_DIR="$HOME/Documents/Data_Database"
;;
# Executable files
apk|bat|cgi|pl|com|exe|gadget|jar|py|wsf)
DEST_DIR="$HOME/Documents/Executable_File"
;;
# Font files
fnt|fon|otf|ttf)
DEST_DIR="$HOME/Documents/Fonts"
;;
# Internet related files
asp|cer|cfm|css|htm|html|js|jsp|php|rss|xhtml)
DEST_DIR="$HOME/Documents/Internet_files"
;;
# Presentation files
key|odp|pps|ppt|pptx)
DEST_DIR="$HOME/Documents/Presentation"
;;
# Programming files
c|class|cpp|cs|h|java|sh|swift|vb)
DEST_DIR="$HOME/Documents/Programming_Files"
;;
# Spreadsheet files
ods|xlr|xls|xlsx)
DEST_DIR="$HOME/Documents/Spreadsheets"
;;
# Anything else
*)
# TODO: handle any unrecognized files here
;;
esac
if [ "$DEST_DIR" = "" ] ; then
# If we didn't find a place for this file, just skip it.
continue
fi
# Now we should have our filename and our destination directory
# So let's do it!
mkdir -p "$DEST_DIR"
chmod +w "$TARGET/$FILENAME"
if ! [ -e "$DEST_DIR/$FILENAME" ] ; then
mv "$TARGET/$FILENAME" "$DEST_DIR"
else
# Don't clobber existing files!
# If we already have a "foo.txt", try "foo.txt.1.txt",
# "foo.txt.2.txt", etc. If we can't find a unique name
# after "foo.txt.99.txt" just give up -- the user can
# deal with it later.
N=0
while [ $N -le 99 ] ; do
if ! [ -e "$DEST_DIR/$FILENAME.$N.$EXT" ] ; then
mv "$TARGET/$FILENAME" "$DEST_DIR/$FILENAME.$N.$EXT"
break # Success!
fi
N=$((N+1))
done
fi
done
답변1
나는 이 문제를 해결했다고 믿습니다. 운 좋게도 이 스크립트의 공동 저자이기도 한 나보다 훨씬 더 재능 있는 Linux 사용자를 만날 수 있었습니다. 그것은 나에게 무례하기 때문에 이름을 밝히지 않을 것입니다. 그러나 그는 나타나서 이것을 추가했습니다. 줄 "[ -s "$TARGET/$FILENAME"] || 계속" "| while read FILENAME; do" 끝 부분에 제가 처음이라서 어떻게 될지 모르겠지만 이제 스크립트는 파일을 다음 이후에 이동할 때까지 기다립니다. 다운로드가 완료되었습니다.
#!/bin/bash
# Requires inotify-tools package
#
# Authors: oddstap && yetanothergeek
#
# This simple tool takes newly created files in the Downloads directory
# and then organizes them based on file extension.
TARGET=$HOME/Downloads
inotifywait -m -e close_write -e moved_to --format "%f" "$TARGET" \
| while read FILENAME; do
[ -s "$TARGET/$FILENAME" ] || continue
EXT=${FILENAME##*.} # Extract file extension
EXT=${EXT,,} # Convert to lowercase
DEST_DIR=''
case "$EXT" in
# Word processor and text files
doc|docx|odt|pdf|rtf|tex|txt|wks|wps|wpd)
DEST_DIR="$HOME/Documents/Word_Processor_And_Text_files"
;;
# Audio files
mp3|wav|wma|mid|midi|aif|cda|mpa|ogg|wpl)
DEST_DIR="$HOME/Music"
;;
# Image files
jpg|jpeg|png|ai|bmp|gif|ico|ps|svg|tif|tiff|psd)
DEST_DIR="$HOME/Pictures"
;;
# Video files
avi|wmv|3g2|3gp|flv|h264|m4v|mkv|mov|mp4|mpg|mpeg|rm|swf|vob|wmv)
DEST_DIR="$HOME/Videos"
;;
# Compressed files
7z|arj|deb|pkg|rar|rpm|gz|z|zip)
DEST_DIR="$HOME/Documents/Compressed_Files"
;;
# Disc and media files
bin|dmg|iso|toast|vcd)
DEST_DIR="$HOME/Documents/Disk_Images"
;;
# Data and database files
csv|dat|db|dbf|log|mdb|sav|sql|tar|xml)
DEST_DIR="$HOME/Documents/Data_Database"
;;
# Executable files
apk|bat|cgi|pl|com|exe|gadget|jar|py|wsf)
DEST_DIR="$HOME/Documents/Executable_File"
;;
# Font files
fnt|fon|otf|ttf)
DEST_DIR="$HOME/Documents/Fonts"
;;
# Internet related files
asp|cer|cfm|css|htm|html|js|jsp|php|rss|xhtml)
DEST_DIR="$HOME/Documents/Internet_files"
;;
# Presentation files
key|odp|pps|ppt|pptx)
DEST_DIR="$HOME/Documents/Presentation"
;;
# Programming files
c|class|cpp|cs|h|java|sh|swift|vb)
DEST_DIR="$HOME/Documents/Programming_Files"
;;
# Spreadsheet files
ods|xlr|xls|xlsx)
DEST_DIR="$HOME/Documents/Spreadsheets"
;;
# Anything else
*)
# TODO: handle any unrecognized files here
;;
esac
if [ "$DEST_DIR" = "" ] ; then
# If we didn't find a place for this file, just skip it.
continue
fi
# Now we should have our filename and our destination directory
# So let's do it!
mkdir -p "$DEST_DIR"
chmod +w "$TARGET/$FILENAME"
if ! [ -e "$DEST_DIR/$FILENAME" ] ; then
mv "$TARGET/$FILENAME" "$DEST_DIR"
else
# Don't clobber existing files!
# If we already have a "foo.txt", try "foo.txt.1.txt",
# "foo.txt.2.txt", etc. If we can't find a unique name
# after "foo.txt.99.txt" just give up -- the user can
# deal with it later.
N=0
while [ $N -le 99 ] ; do
if ! [ -e "$DEST_DIR/$FILENAME.$N.$EXT" ] ; then
mv "$TARGET/$FILENAME" "$DEST_DIR/$FILENAME.$N.$EXT"
break # Success!
fi
N=$((N+1))
done
fi
done