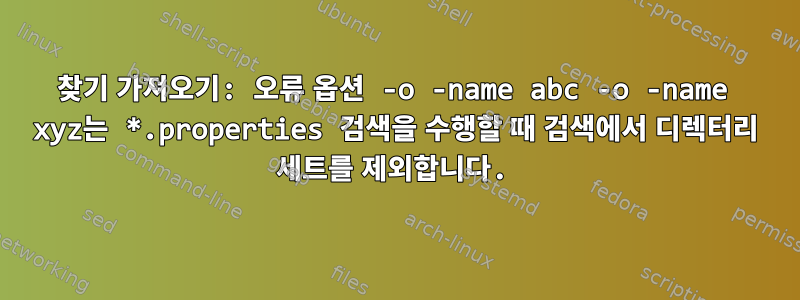
#!/usr/bin/ksh
# *****************************************************************************************
# copy_properties.sh
# This script copies *.properties files from all directory excluding the
# ones provided as the args. to a folder location of our choice.
#
# *****************************************************************************************
echo "Starting the find and replace process for :" "$1"
set -x
# **************** Change Variables Here ************
startdirectory=$2 #"/home/ardsingh/test_properties/properties_files"
destinationFolder=$3
if [ -n "$4" ]; then
listOfFolderTobeIgnored=$4
#@list = split(/ /, $listOfFolderTobeIgnored);
#mapfile -t list << ($listOfFolderTobeIgnored)
IFS=' '
set -A list $listOfFolderTobeIgnored
foldersToBeIgnored="-o -name "${list[0]}
unset 'list[0]'
for item in "${list[@]}"
do
foldersToBeIgnored="$foldersToBeIgnored -o -name "
foldersToBeIgnored="$foldersToBeIgnored$item"
done
else
echo "No input provided for folders to be ignored."
fi
#echo $foldersToBeIgnored
find "$startdirectory" -type d \( -name properties_file_folder_02_25 -o -name brmsdeploy -o -name TempJobs -o -name tmp -o -name logs -o -name deploy "$foldersToBeIgnored" \) -prune -o -name "*.properties" -type f -print -exec cp {} "$destinationFolder" \;
다음 오류가 발생합니다.
find: bad option -o -name abc -o -name xyz
find: [-H | -L] path-list predicate-list
답변1
건물을 짓는 대신끈특정 이름의 디렉터리를 무시하는 명령줄 옵션의 경우 배열을 사용하세요. 이렇게 하면 find
명령줄에서 동적으로 추가하는 옵션이 적절하게 구분됩니다.
다음 스크립트는 단일 검색 경로인 대상 디렉터리와 무시할 디렉터리 이름을 사용하는 매우 기본적인 스크립트입니다. 이 코드는 위치 인수 배열에 대한 find
옵션 세트를 작성합니다 .$@
#!/usr/bin/ksh
searchpath=$1
destdir=$2
shift 2
ignore=( brmsdeploy TempJobs tmp logs deploy )
if [ ! -d "$destdir" ]; then
printf 'Destination directory "%s" does not exist\n' >&2
exit 1
fi
# Process the directories to ignore from the command line.
# This replaces the positional parameters.
for name do
set -- "$@" -o -name "$name"
shift
done
# Process the directories that we always ignore.
# This adds to the positional parameters.
for name in "${ignore[@]}"; do
set -- "$@" -o -name "$name"
done
shift # shift off the first -o
find "$searchpath" \( -type d \( "$@" \) -prune \) -o \
\( -type f -name '*.properties' -exec cp {} "$destdir" \; \)
지침:
./script.sh /path/to/topdir /path/to/destdir somename someothername fluff
이는 및 find
라는 디렉토리를 무시하고 실행 됩니다 somename
. 이를 통해 이름에 공백, 탭 및 개행 문자를 포함할 수도 있습니다(명령줄에서 인용된 경우).someothername
fluff
이 명령 find
으로 실행되는 실제 명령은 다음과 같습니다.
find /path/to/topdir '(' -type d '(' -name somename -o -name someothername -o -name fluff -o -name brmsdeploy -o -name TempJobs -o -name tmp -o -name logs -o -name deploy ')' -prune ')' -o '(' -type f -name '*.properties' -exec cp '{}' /path/to/destdir ';' ')'