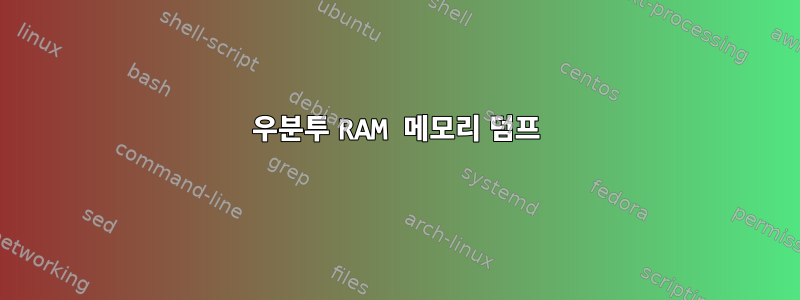
LIME, AVML 등과 같은 도구를 사용하지 않고 pyhton 스크립트를 사용하여 우분투 시스템의 메모리 덤프를 가져오고 싶습니다. gcore와 같은 Linux 내장 명령을 사용하고 싶습니다. 모든 RAM 프로세스의 메모리 덤프를 생성한 후 이러한 메모리 덤프 바이너리의 내용을 사람이 읽을 수 있는 파일로 읽어오고 싶습니다. 루프 장치를 사용하여 이러한 파일을 마운트하려고 시도했지만 "마운트: /mnt: 잘못된 fs 유형, 잘못된 옵션, /dev/loop0의 잘못된 슈퍼 블록, 누락된 코드 페이지 또는 도우미 또는 기타 오류"라는 오류가 표시됩니다. 메모리 덤프를 가져오는 데 사용됩니다.
import subprocess
import os import csv from getpass import getpass
# Check current value of ptrace_scope
result = subprocess.run(['cat', '/proc/sys/kernel/yama/ptrace_scope'], stdout=subprocess.PIPE, text=True) print("Current value of ptrace_scope:", result.stdout)
# If the value is 1 or 2, it means the ptrace_scope is restricted
if result.stdout.strip() in ['1', '2']:
Update ptrace_scope to 0 to allow tracing of all processes
subprocess.run(['sudo', 'tee', '/proc/sys/kernel/yama/ptrace_scope'], input='0', stdout=subprocess.PIPE, text=True)
print("ptrace_scope has been updated to allow tracing of all processes.")
else: print("ptrace_scope is already allowing tracing of all processes.")
try:
Prompt for password to run sudo command
password = input("Enter your password: ")
Use the -S option to read the password from standard input
# Pass the password to sudo command using echo
subprocess.run(['echo', password, '|', 'sudo', '-S', 'chmod', '-R', '777', '/home/memory_dump'], check=True, shell=True) print("File permissions have been updated successfully.") except subprocess.CalledProcessError as e: print(f"Failed to update file permissions. Error: {e}")
def memory_dump(pids, core_dump_dir, password):
Create core dumps for processes with specified PIDs.
# Loop through the PIDs and take core dumps
# Execute ps aux command and capture the output
# Print the list of PIDs
for pid in pids: try:
Execute gcore command to take core dump
subprocess.run(['sudo', '-S', 'gcore', '-o', core_dump_dir, str(pid)], input=password.encode(), check=True) print(f"Core dump for PID {pid} has been successfully created.") except subprocess.CalledProcessError as e: print(f"Failed to create core dump for PID {pid}. Error: {e}")
# Prompt the user to enter the password
password = getpass("Enter your password: ")
# User-specified path to save the CSV file
csv_path = input("Enter the path to save the CSV file (e.g. /path/to/save/): ")
Prompt the user for a filename
filename = input("Enter the filename for the CSV file (e.g. processes.csv): ")
# Join the user-specified path and filename to create the full file path
csv_file_path = os.path.join(csv_path, filename)
# List of PIDs for which core dumps are to be taken
Execute ps aux command and capture the output
ps_output = subprocess.check_output(['ps', 'aux']).decode('utf-8')
# Split the output into lines
ps_lines = ps_output.splitlines()
# Extract the PIDs and process names from the lines
processes = [] for line in ps_lines[1:]:
Skip the first line, which contains the column headers
# Split the line by whitespace
fields = line.split()
The PID is the second field (index 1)
pid = int(fields[1])
The process name is the last field (last element in the list)
process_name = fields[-1]
Add the PID and process name as a tuple to the list of processes
processes.append((pid, process_name))
# Print the list of PIDs and process names
print("List of PIDs and Process Names:") for process in processes: print("PID: {}, Process Name: {}".format(process[0], process[1]))
# Save the list of PIDs and process names to the user-specified CSV file
with open(csv_file_path, 'w', newline='') as csvfile: writer = csv.writer(csvfile) writer.writerow(['PID', 'Process Name']) # Write header row for process in processes: writer.writerow([process[0], process[1]])
Directory to store the core dumps
core_dump_dir = '/home/memory_dump'
Create the core dump directory if it doesn't exist
if not os.path.exists(core_dump_dir): os.makedirs(core_dump_dir)
# Get the list of PIDs from the processes list
pids = [process[0] for process in processes]
# Call the function to create core dumps
memory_dump(pids, core_dump_dir, password)
다음은 코어 덤프 파일을 읽는 데 사용하는 코드입니다.
import subprocess
import os import getpass
def coredump_mount(folder_path, destination_folder_path, sudo_password):
""" Mounts the contents of core dump files in a folder to a loop device and copies the files to a destination folder. """
# Check if the folder path is valid
if not os.path.exists(folder_path): print(f"Folder path {folder_path} does not exist!") exit(1)
# Check if the destination folder path is valid
if not os.path.exists(destination_folder_path): print(f"Destination folder path {destination_folder_path} does not exist!") exit(1)
# Loop through all the files in the folder
file_list = os.listdir(folder_path) for i in range(0, len(file_list), 5): group_files = file_list[i:i+5]
for filename in group_files:
Construct the file path by joining the folder path and the filename
file_path = os.path.join(folder_path, filename)
# Check if the file is a regular file and not a directory
if os.path.isfile(file_path):
Create a loop device with sudo and provide the password
subprocess.run(["sudo", "-S", "losetup", '--force', f"/dev/loop{i%5}", file_path], input=sudo_password, text=True)
# Mount the loop device to a mount point with sudo and provide the password
subprocess.run(["sudo", "-S", "mount", f"/dev/loop{i%5}", "/mnt"], input=sudo_password, text=True)
# Copy files from the loop device to the destination folder with sudo and provide the password
subprocess.run(["sudo", "-S", "cp", "-r", "/mnt/.", destination_folder_path], input=sudo_password, text=True)
# Unmount the loop device with sudo and provide the password
subprocess.run(["sudo", "-S", "mount", "-o", "rw", f"/dev/loop{i%5}", "/mnt"], input=sudo_password, text=True)
# Detach the loop device with sudo and provide the password
subprocess.run(["sudo", "-S", "losetup", "-f", file_path], input=sudo_password, text=True)
# Get folder path from user
folder_path = input("Please enter the folder path containing core dump files: ")
# Get destination folder path from user
destination_folder_path = input("Please enter the destination folder path: ")
# Get sudo password securely from the user
sudo_password = getpass.getpass("Please enter your sudo password: ")
# Call the function with user inputs
coredump_mount(folder_path, destination_folder_path, sudo_password)
코어 덤프 코드를 읽는 동안 오류가 발생했습니다:
"mount: /mnt: wrong fs type, bad option, bad superblock on /dev/loop0, missing codepage or helper program, or other error."
답변1
/dev/loop
메모리는 관련되지 않습니다. 일반 파일을 블록 장치처럼 보이게 만들어 파일 시스템 이미지 및 mount
.
코어 덤프는 파일 시스템과 동일하게 구성되지 않습니다. 그러면 마운트할 수 없습니다.
활성 프로세스의 메모리에 직접 액세스할 수 있지만 /dev/nnn/mem
원하는 /dev/nnn/maps
것이 메모리 구조(논리 페이지가 어떤 파일에 매핑되는지)뿐인 경우에는 이 방법이 편리합니다.