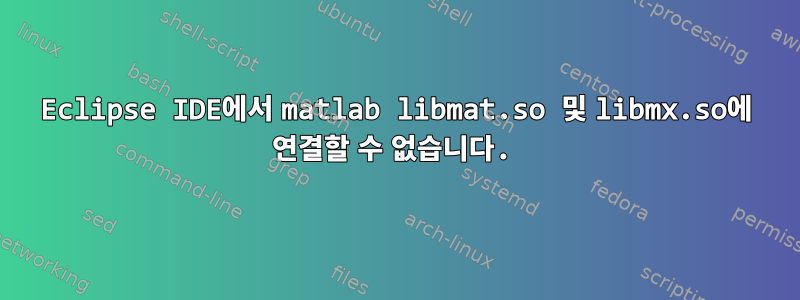
컴파일하려고하는데matcreat.cpp아래에이클립스 C++ IDE. MATLAB 데이터를 C++ 프로그램과 교환할 수 있습니다. 어느 시점에서 나는 프로그램을 컴파일합니다.
/*
* matcreat.cpp - MAT-file creation program
*
* See the MATLAB External Interfaces/API Guide for compiling information.
*
* Calling syntax:
*
* matcreat
*
* Create a MAT-file which can be loaded into MATLAB.
*
* This program demonstrates the use of the following functions:
*
* matClose
* matGetVariable
* matOpen
* matPutVariable
* matPutVariableAsGlobal
*
* Copyright 1984-2007 The MathWorks, Inc.
*/
#include <stdio.h>
#include <string.h> /* For strcmp() */
#include <stdlib.h> /* For EXIT_FAILURE, EXIT_SUCCESS */
#include <vector> /* For STL */
#include "mat.h"
#define BUFSIZE 256
int main() {
MATFile *pmat;
mxArray *pa1, *pa2, *pa3;
std::vector<int> myInts;
myInts.push_back(1);
myInts.push_back(2);
printf("Accessing a STL vector: %d\n", myInts[1]);
double data[9] = { 1.0, 4.0, 7.0, 2.0, 5.0, 8.0, 3.0, 6.0, 9.0 };
const char *file = "mattest.mat";
char str[BUFSIZE];
int status;
printf("Creating file %s...\n\n", file);
pmat = matOpen(file, "w");
if (pmat == NULL) {
printf("Error creating file %s\n", file);
printf("(Do you have write permission in this directory?)\n");
return(EXIT_FAILURE);
}
pa1 = mxCreateDoubleMatrix(3,3,mxREAL);
if (pa1 == NULL) {
printf("%s : Out of memory on line %d\n", __FILE__, __LINE__);
printf("Unable to create mxArray.\n");
return(EXIT_FAILURE);
}
pa2 = mxCreateDoubleMatrix(3,3,mxREAL);
if (pa2 == NULL) {
printf("%s : Out of memory on line %d\n", __FILE__, __LINE__);
printf("Unable to create mxArray.\n");
return(EXIT_FAILURE);
}
memcpy((void *)(mxGetPr(pa2)), (void *)data, sizeof(data));
pa3 = mxCreateString("MATLAB: the language of technical computing");
if (pa3 == NULL) {
printf("%s : Out of memory on line %d\n", __FILE__, __LINE__);
printf("Unable to create string mxArray.\n");
return(EXIT_FAILURE);
}
status = matPutVariable(pmat, "LocalDouble", pa1);
if (status != 0) {
printf("%s : Error using matPutVariable on line %d\n", __FILE__, __LINE__);
return(EXIT_FAILURE);
}
status = matPutVariableAsGlobal(pmat, "GlobalDouble", pa2);
if (status != 0) {
printf("Error using matPutVariableAsGlobal\n");
return(EXIT_FAILURE);
}
status = matPutVariable(pmat, "LocalString", pa3);
if (status != 0) {
printf("%s : Error using matPutVariable on line %d\n", __FILE__, __LINE__);
return(EXIT_FAILURE);
}
/*
* Ooops! we need to copy data before writing the array. (Well,
* ok, this was really intentional.) This demonstrates that
* matPutVariable will overwrite an existing array in a MAT-file.
*/
memcpy((void *)(mxGetPr(pa1)), (void *)data, sizeof(data));
status = matPutVariable(pmat, "LocalDouble", pa1);
if (status != 0) {
printf("%s : Error using matPutVariable on line %d\n", __FILE__, __LINE__);
return(EXIT_FAILURE);
}
/* clean up */
mxDestroyArray(pa1);
mxDestroyArray(pa2);
mxDestroyArray(pa3);
if (matClose(pmat) != 0) {
printf("Error closing file %s\n",file);
return(EXIT_FAILURE);
}
/*
* Re-open file and verify its contents with matGetVariable
*/
pmat = matOpen(file, "r");
if (pmat == NULL) {
printf("Error reopening file %s\n", file);
return(EXIT_FAILURE);
}
/*
* Read in each array we just wrote
*/
pa1 = matGetVariable(pmat, "LocalDouble");
if (pa1 == NULL) {
printf("Error reading existing matrix LocalDouble\n");
return(EXIT_FAILURE);
}
if (mxGetNumberOfDimensions(pa1) != 2) {
printf("Error saving matrix: result does not have two dimensions\n");
return(EXIT_FAILURE);
}
pa2 = matGetVariable(pmat, "GlobalDouble");
if (pa2 == NULL) {
printf("Error reading existing matrix GlobalDouble\n");
return(EXIT_FAILURE);
}
if (!(mxIsFromGlobalWS(pa2))) {
printf("Error saving global matrix: result is not global\n");
return(EXIT_FAILURE);
}
pa3 = matGetVariable(pmat, "LocalString");
if (pa3 == NULL) {
printf("Error reading existing matrix LocalString\n");
return(EXIT_FAILURE);
}
status = mxGetString(pa3, str, sizeof(str));
if(status != 0) {
printf("Not enough space. String is truncated.");
return(EXIT_FAILURE);
}
if (strcmp(str, "MATLAB: the language of technical computing")) {
printf("Error saving string: result has incorrect contents\n");
return(EXIT_FAILURE);
}
/* clean up before exit */
mxDestroyArray(pa1);
mxDestroyArray(pa2);
mxDestroyArray(pa3);
if (matClose(pmat) != 0) {
printf("Error closing file %s\n",file);
return(EXIT_FAILURE);
}
printf("Done\n");
return(EXIT_SUCCESS);
}
어느 순간 프로그램이 libmat.so와 libmx.so Matlab API에 의존하게 되는데, Eclipse에서 어떻게 링크하는지 알고 싶습니다. 어떤 링크도 없이 나는 얻는다
18:57:21 **** Incremental Build of configuration Debug for project essai ****
make all
Building target: essai
Invoking: GCC C++ Linker
g++ -o "essai" ./matcreat.o
/usr/bin/ld : ./matcreat.o : dans la fonction « main » :
/home/baptiste/Code_Info/PLPLP4/PLPLP4_DEV/essai/Debug/../matcreat.cpp:44 : référence indéfinie vers « matOpen_800 »
/usr/bin/ld : /home/baptiste/Code_Info/PLPLP4/PLPLP4_DEV/essai/Debug/../matcreat.cpp:51 : référence indéfinie vers « mxCreateDoubleMatrix_800 »
/usr/bin/ld : /home/baptiste/Code_Info/PLPLP4/PLPLP4_DEV/essai/Debug/../matcreat.cpp:58 : référence indéfinie vers « mxCreateDoubleMatrix_800 »
/usr/bin/ld : /home/baptiste/Code_Info/PLPLP4/PLPLP4_DEV/essai/Debug/../matcreat.cpp:64 : référence indéfinie vers « mxGetPr_800 »
/usr/bin/ld : /home/baptiste/Code_Info/PLPLP4/PLPLP4_DEV/essai/Debug/../matcreat.cpp:66 : référence indéfinie vers « mxCreateString_800 »
/usr/bin/ld : /home/baptiste/Code_Info/PLPLP4/PLPLP4_DEV/essai/Debug/../matcreat.cpp:73 : référence indéfinie vers « matPutVariable_800 »
/usr/bin/ld : /home/baptiste/Code_Info/PLPLP4/PLPLP4_DEV/essai/Debug/../matcreat.cpp:79 : référence indéfinie vers « matPutVariableAsGlobal_800 »
/usr/bin/ld : /home/baptiste/Code_Info/PLPLP4/PLPLP4_DEV/essai/Debug/../matcreat.cpp:85 : référence indéfinie vers « matPutVariable_800 »
/usr/bin/ld : /home/baptiste/Code_Info/PLPLP4/PLPLP4_DEV/essai/Debug/../matcreat.cpp:96 : référence indéfinie vers « mxGetPr_800 »
/usr/bin/ld : /home/baptiste/Code_Info/PLPLP4/PLPLP4_DEV/essai/Debug/../matcreat.cpp:97 : référence indéfinie vers « matPutVariable_800 »
/usr/bin/ld : /home/baptiste/Code_Info/PLPLP4/PLPLP4_DEV/essai/Debug/../matcreat.cpp:104 : référence indéfinie vers « mxDestroyArray_800 »
/usr/bin/ld : /home/baptiste/Code_Info/PLPLP4/PLPLP4_DEV/essai/Debug/../matcreat.cpp:105 : référence indéfinie vers « mxDestroyArray_800 »
/usr/bin/ld : /home/baptiste/Code_Info/PLPLP4/PLPLP4_DEV/essai/Debug/../matcreat.cpp:106 : référence indéfinie vers « mxDestroyArray_800 »
/usr/bin/ld : /home/baptiste/Code_Info/PLPLP4/PLPLP4_DEV/essai/Debug/../matcreat.cpp:108 : référence indéfinie vers « matClose_800 »
/usr/bin/ld : /home/baptiste/Code_Info/PLPLP4/PLPLP4_DEV/essai/Debug/../matcreat.cpp:116 : référence indéfinie vers « matOpen_800 »
/usr/bin/ld : /home/baptiste/Code_Info/PLPLP4/PLPLP4_DEV/essai/Debug/../matcreat.cpp:125 : référence indéfinie vers « matGetVariable_800 »
/usr/bin/ld : /home/baptiste/Code_Info/PLPLP4/PLPLP4_DEV/essai/Debug/../matcreat.cpp:130 : référence indéfinie vers « mxGetNumberOfDimensions_800 »
/usr/bin/ld : /home/baptiste/Code_Info/PLPLP4/PLPLP4_DEV/essai/Debug/../matcreat.cpp:135 : référence indéfinie vers « matGetVariable_800 »
/usr/bin/ld : /home/baptiste/Code_Info/PLPLP4/PLPLP4_DEV/essai/Debug/../matcreat.cpp:140 : référence indéfinie vers « mxIsFromGlobalWS_800 »
/usr/bin/ld : /home/baptiste/Code_Info/PLPLP4/PLPLP4_DEV/essai/Debug/../matcreat.cpp:145 : référence indéfinie vers « matGetVariable_800 »
/usr/bin/ld : /home/baptiste/Code_Info/PLPLP4/PLPLP4_DEV/essai/Debug/../matcreat.cpp:151 : référence indéfinie vers « mxGetString_800 »
/usr/bin/ld : /home/baptiste/Code_Info/PLPLP4/PLPLP4_DEV/essai/Debug/../matcreat.cpp:162 : référence indéfinie vers « mxDestroyArray_800 »
/usr/bin/ld : /home/baptiste/Code_Info/PLPLP4/PLPLP4_DEV/essai/Debug/../matcreat.cpp:163 : référence indéfinie vers « mxDestroyArray_800 »
/usr/bin/ld : /home/baptiste/Code_Info/PLPLP4/PLPLP4_DEV/essai/Debug/../matcreat.cpp:164 : référence indéfinie vers « mxDestroyArray_800 »
/usr/bin/ld : /home/baptiste/Code_Info/PLPLP4/PLPLP4_DEV/essai/Debug/../matcreat.cpp:166 : référence indéfinie vers « matClose_800 »
collect2: erreur: ld a retourné le statut de sortie 1
make: *** [makefile:60 : essai] Erreur 1
"make all" terminated with exit code 2. Build might be incomplete.
그래서 나도 따라해보려고https://stackoverflow.com/questions/61220237/undefine-references-for-mat-h-in-c-l/usr/local/MATLAB/R2020b/bin/glnxa64/libmx.so -l/usr/local/MATLAB/R2020b/bin/glnxa64/libmat.so를 팝퍼티 >> C++ 빌드 >> 설정 >>GCC C++에 넣습니다. 링커 >> 링커 플래그.
알겠어요
19:00:44 **** Incremental Build of configuration Debug for project essai ****
make all
Building target: essai
Invoking: GCC C++ Linker
g++ -l/usr/local/MATLAB/R2020b/bin/glnxa64/libmx.so -l/usr/local/MATLAB/R2020b/bin/glnxa64/libmat.so -o "essai" ./matcreat.o
/usr/bin/ld : ne peut trouver -l/usr/local/MATLAB/R2020b/bin/glnxa64/libmx.so
/usr/bin/ld : ne peut trouver -l/usr/local/MATLAB/R2020b/bin/glnxa64/libmat.so
collect2: erreur: ld a retourné le statut de sortie 1
make: *** [makefile:60 : essai] Erreur 1
"make all" terminated with exit code 2. Build might be incomplete
어떤 아이디어가 있나요? 저는 C++을 처음 접했습니다. 미리 감사드립니다. B
답변1
ldd
컴파일러 명령이 아닙니다. 일부 실행 파일(또는 다른 동적 라이브러리)에 필요한 동적 라이브러리 목록을 제공하는 도구입니다.
컴파일러의 명령줄에서 제거하십시오.
먼저 소스 코드를 개체 파일로 컴파일합니다( -c
컴파일러 명령줄에 입력). 결과는 matcreat.o(key) 파일에 있습니다 -o "matcreat.o"
.
이 파일(및 다른 파일)은 실행 파일 또는 동적 라이브러리를 빌드하기 위해 연결됩니다(명령은 게시물에 없으므로 이에 대해 아무 것도 알 수 없음).
ldd -d /usr/local/MATLAB/R2020b/bin/glnxa64/libmat.so
동적 라이브러리에서 사용하는 라이브러리 목록을 제공합니다 libmat.so
. 해당 라이브러리가 없으면 라이브러리 자체가 작동하지 않습니다.
자신의 실행 파일(또는 라이브러리)을 성공적으로 컴파일하고 libmat.so에 연결하면 이것이 ldd myexe
표시됩니다 libmat.so
(그러나 libmat.so에서 사용하는 라이브러리는 표시되지 않음).