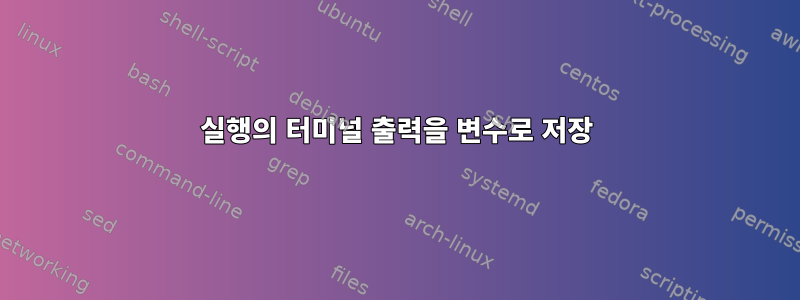
package.json 을 사용하는 Python 스크립트를 실행 중입니다 pyserial
. 보드를 사용하여 모터 회전을 제어하고 USB 포트를 통해 연결합니다.
보드는 주어진 명령에 따라 모터를 회전하도록 프로그래밍되었습니다. 여기 몇 가지 예가 있어요.
- 입력 : 모터 상태 확인 명령어 : H010100
산출:
{
.."beambreak": [0,0,0,0,0],
.."prox": [0.003,0.003],
.."T1": [0,0],
.."T2": [0,0],
.."MT": [-1,-1]
.."test_switch": 0,
.}
- 입력 : 모터 1회전 지령 : H010101
출력: {"회전":"성공"}
일: "while" 루프에서 1분마다 명령(예: H010101)을 보내는 방법, 출력 메시지(예: {"Rotate": "Successful"})를 확인하고 출력 메시지 조건에 따라 다음 명령을 보내는 방법입니다.
질문: 코드를 실행하면 Linux 터미널/IDE 콘솔에 "설정 가능" 출력 메시지가 나타나는데, 메시지를 변수로 저장하고 루프 조건에 적용하는 방법을 모르겠습니다. 즉, 메시지가 동일한 메시지인지 확인하고 1분 정도 기다린 후 H010101 명령을 다시 보내시겠습니까?
또한 *.log 또는 *.txt에 파일을 저장하려고 시도했지만 작동하지 않습니다. 예:
$ python test.py >> *.txt
$ python test.py > *.log
이것은 내 코드입니다.
import time
import serial
# configure the serial connections
ser = serial.Serial(
port='/dev/ttyUSB0',
baudrate=115200,
parity=serial.PARITY_NONE,
stopbits=serial.STOPBITS_ONE,
bytesize=serial.EIGHTBITS
)
while True :
print(' Check Status')
ser.write('H010000\n'.encode())
status_cmd = ser.readline()
print(status_cmd)
if status_cmd === "test_switch: 0 " : # i can't save the message as variable from running terminal
time.sleep(5)
# Command to rotate motor
ser.write('H010101\n'.encode())
# read respond of give command
reading = ser.readline()
print(reading)
if reading == {"Drop":"Successful"} : # i can't save the message as variable from running terminal
time.sleep(60)
# rotate motor
ser.write('H010101\n'.encode())
# read respond of give command
reading = ser.readline()
print(reading)
답변1
가장 먼저 할 수 있는 일은 함수를 메소드로 캡슐화하는 것입니다(또는 원하는 경우 클래스를 사용하는 것).
상태 확인
def check_status(ser):
print('Check Status')
ser.write('H010000\n'.encode())
current_status = ser.readline().decode('utf-8')
return current_status
회전 모터
def rotate_motor(ser):
print('Rotating ..')
ser.write('H010101\n'.encode())
rotation_status = ser.readline().decode('utf-8')
return rotation_status
또한 응답을 dict로 로드하려면 json을 가져와야 합니다.
예를 들어
>>> import json
>>> rotation_status='{"Drop":"Successful"}'
>>> json.loads(rotation_status)
{'Drop': 'Successful'}
이제 이러한 코드 조각이 준비되었으므로 이를 호출하여 결과에 따라 지속적으로 작업을 실행할 수 있습니다.
while True:
status = json.loads(check_status(ser))
if status['test_switch'] == 0:
time.sleep(5)
is_rotated = json.loads(rotate_motor(ser))
if is_rotated['Drop'] == 'Successful':
time.sleep(60)
else:
print('Something went wrong')
raise Exception(is_rotated)