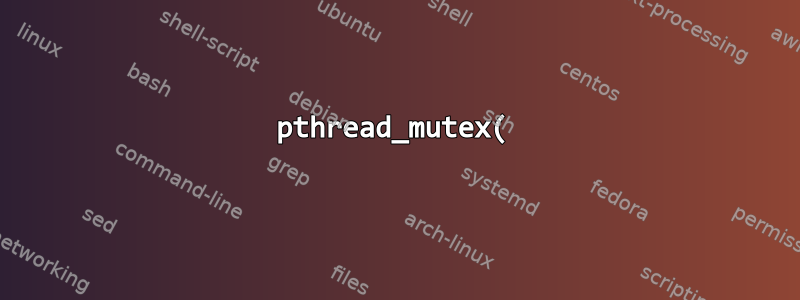%EA%B0%80%20%EC%8B%A4%ED%96%89%EB%90%A0%20%EB%95%8C%20%ED%94%84%EB%A1%9C%EC%84%B8%EC%8A%A4%20%EC%83%81%ED%83%9C%EB%8A%94%20%EB%AC%B4%EC%97%87%EC%9E%85%EB%8B%88%EA%B9%8C%3F.png)
다음은 Linux 프로세스 상태입니다.
R: running or runnable, it is just waiting for the CPU to process it
S: Interruptible sleep, waiting for an event to complete, such as input from the terminal
D: Uninterruptible sleep, processes that cannot be killed or interrupted with a signal, usually to make them go away you have to reboot or fix the issue
Z: Zombie, we discussed in a previous lesson that zombies are terminated processes that are waiting to have their statuses collected
T: Stopped, a process that has been suspended/stopped
두 가지 질문이 있습니다.
(1) 프로세스/스레드에서 mutex_lock()이 호출될 때 잠금이 획득되기를 기다리고 있는 경우(다른 스레드가 이미 뮤텍스 잠금을 잠근 경우) 프로세스는 S
또는 로 이동합니까 D
?
(2) 나는 spinlock()이 프로세스를 바쁜 대기 상태로 만들고 다른 스레드에 의해 잠금이 해제되었는지 확인한다는 것을 알고 있습니다. 그러나 mutex_lock()에서는 잠금이 해제되어 잠기고 계속할 수 있다는 것을 어떻게 알 수 있습니까? IE; sleep
뮤텍스가 사용 가능하면(다른 스레드에 의해 잠금 해제됨) 잠긴 뮤텍스에서 프로세스가 어떻게 깨어납니까?
답변1
글쎄요, 알아봅시다:
#include <pthread.h>
#include <sys/types.h>
#include <unistd.h>
#include <stdio.h>
int main ()
{
pthread_mutex_t mtx;
pid_t pid;
pthread_mutex_init (&mtx, NULL);
pid = getpid();
printf ("pid : %d\n", pid);
pthread_mutex_lock (&mtx);
// Double lock. This results in a dead-lock
pthread_mutex_lock (&mtx);
pthread_mutex_destroy (&mtx);
}
엮다:
gcc -lpthread -o prog prog.c
그런 다음 다음을 실행하십시오.
./prog
pid : 23537
(이것은 내 구체적인 경우의 pid입니다. 실행하면 다른 결과가 발생합니다)
이제 이 프로세스의 상태를 살펴보겠습니다.
ps aux | grep 23537
user 23537 0.0 0.0 6448 840 pts/4 S+ 16:29 0:00 ./prog
이것S+프로세스의 상태입니다.
매뉴얼 페이지에 따르면
PROCESS STATE CODES
Here are the different values that the s, stat and state output specifiers (header "STAT" or "S") will display to describe the state of a
process:
D uninterruptible sleep (usually IO)
R running or runnable (on run queue)
S interruptible sleep (waiting for an event to complete)
T stopped by job control signal
t stopped by debugger during the tracing
W paging (not valid since the 2.6.xx kernel)
X dead (should never be seen)
Z defunct ("zombie") process, terminated but not reaped by its parent
For BSD formats and when the stat keyword is used, additional characters may be displayed:
< high-priority (not nice to other users)
N low-priority (nice to other users)
L has pages locked into memory (for real-time and custom IO)
s is a session leader
l is multi-threaded (using CLONE_THREAD, like NPTL pthreads do)
+ is in the foreground process group
알겠어요포그라운드 프로세스 그룹에서 중단 가능한 절전 모드(이벤트가 완료될 때까지 대기)
두 번째 질문에 관해서: 스레드가 잠긴 뮤텍스를 획득하려고 시도하면 커널은 CPU에서 스레드를 제거하고 대기열에 저장합니다. 소유자가 잠금을 해제하면 커널은 대기열에 스레드가 있는지 확인하고 해당 스레드를 깨웁니다(아마도).
이는 스핀 잠금과 다릅니다. 스핀 잠금은 다음과 같습니다.
int lock = 1;
// 1 means locked, 0 means unlocked
while (lock) {};
// If we are here, it means the lock somehow changed to 0
lock = 1;
// We set it to one again to avoid another thread taking it
그러나 이 구현은 원자적이지 않습니다. 커널은 이를 원자적으로 수행하기 위한 몇 가지 방법을 제공합니다.