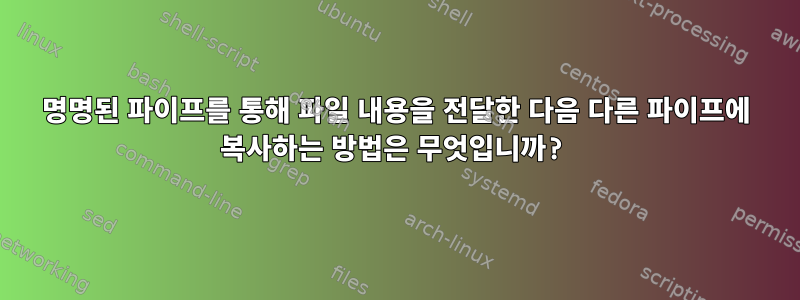
나는 2개의 프로그램을 작성했습니다. 일반적으로 1. 명명된 파이프(서버)를 생성하고 2. 셸에서 서버 부분으로 문자열을 전달합니다.
터미널에서 명명된 파이프의 서버 부분으로 문자열을 전달하는 방법을 이해합니다. 그러나 파일 내용의 출력을 읽고 다른 파일(아마도 명명된 파이프의 서버 부분)에 전달할 수 있도록 파일을 2개 프로그램에 매개변수로 전달하는 방법을 모르겠습니다. 아이디어는 첫 번째 파일의 내용을 두 번째 파일에 복사하는 것입니다. 불행히도 이것을 구현하는 방법을 모르겠습니다.
#include <stdio.h>
#include <sys/stat.h>
#include <string.h>
#include <stdlib.h>
#include <fcntl.h>
#define FIFO_NAME "myfifo"
#define BUF_SIZE 512
int main (void)
{
FILE * fifo;
char * buf;
if (mkfifo ("myfifo", 0640) == -1) {
fprintf (stderr, "Can't create fifo\n");
return 1;
}
fifo = fopen (FIFO_NAME, "r");
if (fifo == NULL) {
fprintf (stderr, "Cannot open fifo\n");
return 1;
}
buf = (char *) malloc (BUF_SIZE);
if (buf == NULL) {
fprintf (stderr, "malloc () error\n");
return 1;
}
fscanf (fifo, "%s", buf);
printf ("%s\n", buf);
fclose (fifo);
free (buf);
unlink (FIFO_NAME);
return 0;
}
#include <stdio.h>
#include <fcntl.h>
#include <string.h>
#include <stdlib.h>
#include <sys/stat.h>
#define FIFO_NAME "myfifo"
int main (int argc, char ** argv)
{
int fifo;
if (argc < 2) {
fprintf (stderr, "Too few arguments\n");
return 1;
}
fifo = open (FIFO_NAME, O_WRONLY);
if (fifo == -1) {
fprintf (stderr, "Cannot open fifo\n");
return 1;
}
if (write (fifo, argv[1], strlen (argv[1])) == -1) {
fprintf (stderr, "write() error\n");
return 1;
}
close (fifo);
return 0;
}
답변1
나는 당신이 묻는 것을 완전히 이해하지 못합니다. 내 해석은 명령줄에서 클라이언트에 제공된 메시지를 보내는 것이 아니라 파일 이름을 지정하고 해당 파일의 내용을 서버로 파이프하기를 원한다는 것입니다. 그렇다면 다음과 같은 방법이 도움이 될 수 있습니다.
서버는 다음과 같습니다.
#include <stdio.h>
#include <sys/stat.h>
#include <stdlib.h>
#include <unistd.h>
#define FIFO_NAME "myfifo"
#define BUF_SIZE 512
int main(void)
{
if (mkfifo("myfifo", 0640) == -1) {
fprintf(stderr, "Can't create fifo\n");
return EXIT_FAILURE;
}
FILE *fifo = fopen(FIFO_NAME, "r");
if (fifo == NULL) {
fprintf(stderr, "Cannot open fifo\n");
return EXIT_FAILURE;
}
char *line = NULL;
size_t line_length = 0;
while (getline(&line, &line_length, fifo) != EOF) {
printf("%s", line);
}
free(line);
fclose(fifo);
unlink(FIFO_NAME);
return EXIT_SUCCESS;
}
클라이언트는 다음과 같습니다.
#include <stdio.h>
#include <fcntl.h>
#include <stdlib.h>
#include <unistd.h>
#define FIFO_NAME "myfifo"
int main(int argc, char **argv)
{
if (argc < 2) {
fprintf(stderr, "Too few arguments\n");
return EXIT_FAILURE;
}
const int input_fd = open(argv[1], O_RDONLY);
if (input_fd < 0) {
perror("open");
}
const int fifo_fd = open(FIFO_NAME, O_WRONLY);
if (fifo_fd < 0) {
perror("open");
return EXIT_FAILURE;
}
char buffer[4096];
int bytes_used;
while ((bytes_used = read(input_fd, buffer, sizeof(buffer))) > 0) {
if (write(fifo_fd, buffer, bytes_used) < 0) {
perror("write");
return EXIT_FAILURE;
}
}
close(fifo_fd);
close(input_fd);
return EXIT_SUCCESS;
}
이는 클라이언트가
- 명령줄 옵션을 파일 이름으로 사용
- 파일 열기
- 루프에서 파일을 읽고 파이프에 씁니다.
마찬가지로 파이프에서 여러 문자열을 읽을 수 있도록 서버를 수정했습니다. 현재는 표준 출력에서 읽은 모든 내용을 인쇄합니다. 예제 실행을 고려해보세요:
먼저 서버를 시작합니다.
$ gcc -o server server.c
$ ./server
다음으로 입력 파일을 사용하여 클라이언트를 실행합니다.
$ cat message
this is a file
it has multiple lines
$ ./client message
그러면 서버에서 다음 출력이 표시됩니다.
this is a file
it has multiple lines
stdout 대신 파일에 쓰려는 경우 이는 매우 간단한 변경일 수 있습니다.