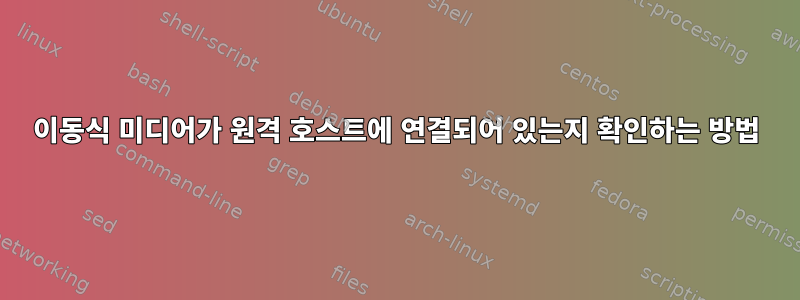
내 네트워크나 서브넷에 USB나 이동식 미디어가 연결되어 있는지 확인해야 합니다. 지금 내가 하고 있는 일은 lsusb 또는 다른 명령을 실행하여 간단한 클라이언트/서버 모델을 만들고, 내가 원하는 클라이언트 USB 정보로 서로 메시지를 주고받은 다음 명령 출력을 서버로 보내는 것입니다. 어떻게 이를 달성할 수 있습니까? 아니면 더 좋은 방법이 있습니까? 저는 Linux를 클라이언트/서버로 사용하고 있습니다. 이것은 내 클라이언트 코드입니다. 클라이언트가 로그를 서버로 보내도록 변경했습니다.
import socket
import threading
import sys
#Wait for incoming data from server
#.decode is used to turn the message in bytes to a string
def receive(socket, signal):
while signal:
try:
data = socket.recv(32)
print(str(data.decode("utf-8")))
except:
print("You have been disconnected from the server")
signal = False
break
#Get host and port
host = input("Host: ")
port = int(input("Port: "))
#Attempt connection to server
try:
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
sock.connect((host, port))
except:
print("Could not make a connection to the server")
input("Press enter to quit")
sys.exit(0)
#Create new thread to wait for data
receiveThread = threading.Thread(target = receive, args = (sock, True))
receiveThread.start()
#Send data to server
#str.encode is used to turn the string message into bytes so it can be sent across the network
while True:
message = input()
sock.sendall(str.encode(message))