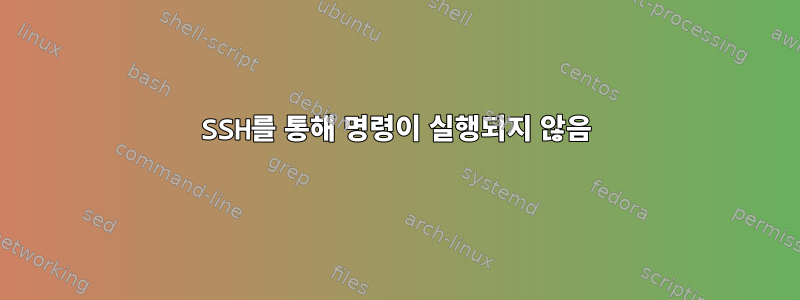
나는 이 강좌를 위해 이 스크립트를 만들었습니다. 스크립트 인수의 파일에 지정된 여러 원격 서버에서 ssh를 통해 명령을 실행합니다.
#!/bin/bash
# The server file. Can be changed with the -f argument
SERVER_FILE='/vagrant/servers'
# The function to check if the chosen SERVER_FILE exists
filecheck() {
if [[ ! -e $SERVER_FILE ]]; then
echo "The file $SERVER_FILE does not exist." >&2
exit 1
fi
}
# The usage statement
usage() {
echo "usage $0 -vsn -f FILE 'COMMAND'"
echo " -v Verbose mode"
echo " -s Run command as sudo on remote server"
echo " -n Dry run, commands not actually executed"
echo " -f FILE Selects a different file other than /vagrant/servers"
exit 1
}
# The verbose mode text things
say() {
if [[ $VERBOSE = 'true' ]]; then
echo "$@"
fi
}
# The ssh command
sshing() {
ssh -o ConnectTimeout=2 $SERVER $@
}
# User executing the command should not be root
if [[ $UID -eq 0 ]]; then
echo "You should not execute this script with sudo or as root" >&2
echo "Use the -s argument if you want sudo powers" >&2
exit 1
fi
# DRYMODE is sshing by Default
DRYMODE='sshing'
#check to see if file SERVER_FILE exists
filecheck
# The options for the script
while getopts vsnf: OPTION; do
case $OPTION in
v)
echo "Verbose mode on"
VERBOSE='true'
;;
s)
say "Sudo mode"
SUDO='sudo'
;;
n)
say "Dry run mode"
DRYMODE='echo'
DRYRUN='DRY RUN: '
echo "DRY RUN MODE ON: "
echo
;;
f)
say "Different file mode"
SERVER_FILE=${OPTARG}
#check to see if file SERVER_FILE exists
filecheck
;;
*)
usage
;;
esac
done
echo
# shifts so that the options are removed from the list of arguments
shift $((OPTIND-1))
#Set a variable for the rest of the arguments, as a command
COMMAND="${@}"
# Checks if the user provided any arguments apart from the optinos
if [[ $# -eq 0 ]]; then
usage
exit 1
fi
# Executes the commands
for SERVER in $(cat ${SERVER_FILE}); do
say "Executing ${COMMAND} on ${SERVER}:"
$DRYMODE $DRYRUN $SUDO ${COMMAND} 2> /dev/null
CMDEX=$?
# if the exit status is 255, something is wrong with the server or is unreachable
if [[ $CMDEX -eq 255 ]]; then
echo "The server you're trying to reach does not exist or is unreachable. Aborting." >&2
exit 1
fi
# if the exit status is non 0 and non 255, something is wrong with the command
if [[ $CMDEX -ne 0 ]]; then
echo "Invalid command ${COMMAND} or wrong syntax. Aborting." >&2
exit 1
# if the exit status is non 0 and non 255, something is wrong with the command
fi
say "Command ${COMMAND} executed successfuly."
done
exit 0
간단한 명령(예: ls
even ps
) 에서는 adduser test
완벽하게 작동 하지만, 큰따옴표가 포함된 명령을 주면 전체 명령을 작은따옴표로 묶지 않으면 작동이 중단됩니다.
이제 이것이 내 코드의 버그인지 아니면 다른 문제인지는 알 수 없지만 이를 통해 명령을 전달할 수는 없습니다.
따라서 이 명령은 작동하지 않습니다.
[vagrant@admin01 vagrant]$ ./run-everywhere.sh -sv 'echo 1 | passwd --stdin test4'
\|로 파이프를 탈출하면 문자 그대로 \|로 쓰여집니다. 이 다른 명령도 작동하지 않습니다.
[vagrant@admin01 vagrant]$ ./run-everywhere.sh -sv 'echo "1" | sha256sum > file1'
편집하다:
파이프가 작동하지 않을 때 발견한 문제: 명령에 sudo 권한이 필요한 경우 파이프 뒤에 sudo도 작성해야 합니다. 이것이 작동하는 방식입니다:
[vagrant@admin01 vagrant]$ ./run-everywhere.sh -sv 'echo 1 | sudo passwd --stdin test4'
하지만 여전히 리디렉션할 수 없습니다.
답변1
이 시도:
sshing () {
ssh -o ConnectTimeout=2 "$SERVER" "$@"
# ................................^..^ crucial quotes
}
# ...
cmd="$*"
# ...
while read -r SERVER; do
say "Executing ${COMMAND} on ${SERVER}:"
$DRYMODE $DRYRUN $SUDO sh -c "${COMMAND}" 2> /dev/null
# .....................11111.2..........2
# 1. run with a shell to enable redirections and pipe
# 2. crucial quotes
# ...
done < "$SERVER_FILE"
sudo를 사용하여 셸에서 명령을 실행하면 전체 파이프라인이 높은 권한으로 실행될 수 있습니다.
또한 변수 이름을 모두 대문자로 사용하는 습관을 버려야 합니다. 어느 날 실수로 PATH를 덮어쓰고 스크립트가 왜 손상되었는지 궁금해하게 될 것입니다.
답변2
문제가 발견되었습니다. 일부 파일을 자체적으로 처리하기 위해 sudo 권한이 있는 스크립트를 실행하면 루트 이름과 그룹 아래에 파일이 생성됩니다. 이는 파일에 대한 권한이 없음을 의미합니다.