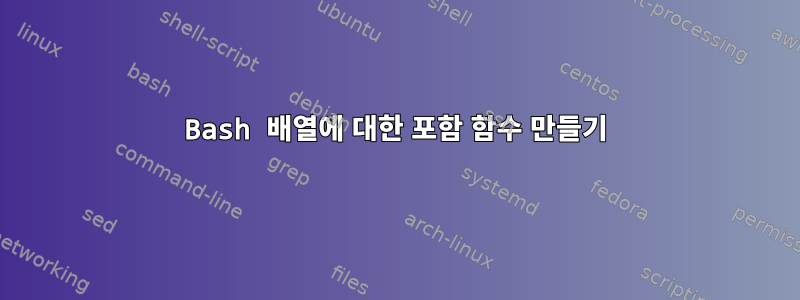
배열에 특정 값이 있는지 확인하는 함수가 포함되어 있습니다. 배열 자체는 첫 번째 매개변수로 전달되고 값은 두 번째 매개변수로 전달됩니다.
#!/usr/bin/env bash
set -e;
branch_type="${1:-feature}";
arr=( 'feature', 'bugfix', 'release' );
contains() {
local array="$1"
local seeking="$2"
echo "seeking => $seeking";
# for v in "${!array}"; do
for v in "${array[@]}"; do
echo "v is $v";
if [ "$v" == "$seeking" ]; then
echo "v is seeking";
return 0;
fi
done
echo "returning with 1";
return 1;
}
if ! contains "$arr" "$branch_type"; then
echo "Branch type needs to be either 'feature', 'bugfix' or 'release'."
echo "The branch type you passed was: $branch_type"
exit 1;
fi
echo "all goode. branch type is $branch_type";
인수 없이 스크립트를 실행하면 기본값이 "feature"이므로 작동하지만 어떤 이유로 검색 결과가 일치하지 않습니다. 오류는 발생하지 않지만 포함 기능이 예상대로 작동하지 않습니다.
매개변수 없이 스크립트를 실행하면 다음과 같은 결과가 나타납니다.
seeking => feature
v is feature,
returning with 1
Branch type needs to be either 'feature', 'bugfix' or 'release'.
The branch type you passed was: feature
이제 이거 이상해
답변1
노트:Bash 4에서 작동하도록 이 문제를 해결하는 방법을 보여 드리겠습니다.
배열을 함수에 전달하는 방식이 잘못된 것 같습니다.
$ cat contains.bash
#!/usr/bin/env bash
branch_type="${1:-feature}";
arr=('feature' 'bugfix' 'release');
contains() {
local array=$1
local seeking="$2"
for v in ${!array}; do
if [ "$v" == "$seeking" ]; then
return 0;
fi
done
return 1;
}
if ! contains arr $branch_type; then
echo "Branch type needs to be either 'feature', 'bugfix' or 'release'."
echo "The branch type you passed was: $branch_type"
exit 1;
fi
echo "the array contains: $branch_type";
나는 상황을 조금 바꾸었고 지금은 작동하는 것 같습니다.
$ ./contains.bash
the array contains: feature
다양성
원본 스크립트에서 두 가지만 변경했습니다. 다음 줄을 사용하여 배열의 기본 이름을 contains()
전달하도록 함수가 호출되는 방식을 변경했습니다 .arr
if ! contains arr $branch_type; then
contains()
그리고 전달된 매개변수로 배열을 설정하는 함수 내에서 다음 줄을 변경하여 지역 변수 설정에서 따옴표를 제거했습니다 array
.
local array=$1
인용하다
답변2
Bash3 관용구는 다음과 같습니다:
#!/usr/bin/env bash
set -e;
branch_type="${1:-feature}";
arr=( 'feature' 'bugfix' 'release' );
contains() {
local seeking="$1"
shift 1;
local arr=( "$@" )
for v in "${arr[@]}"; do
if [ "$v" == "$seeking" ]; then
return 0;
fi
done
return 1;
}
if ! contains "$branch_type" "${arr[@]}"; then
echo "Branch type needs to be either 'feature', 'bugfix' or 'release'."
echo "The branch type you passed was: $branch_type"
exit 1;
fi
echo "the array contains: $branch_type";
진짜 끊김은 내가 이것을 하려고 할 때이다:
local seeking="$1"
local arr=( "$2" )
그러나 이것은 필요합니다:
local seeking="$1"
shift 1;
local arr=( "$@" )