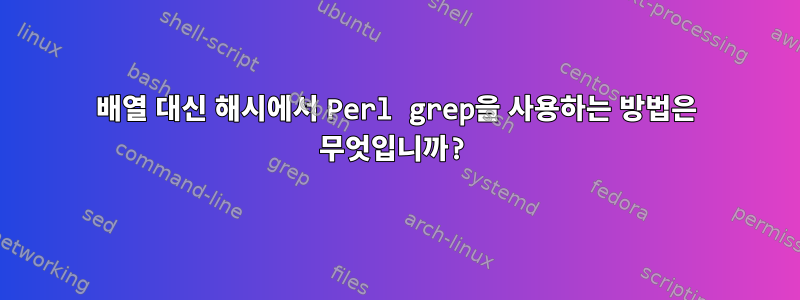
저는 펄을 배우고 있어요. grep
배열에서 사용할 수 있었는데 구문은 다음과 같이 매우 간단합니다.예:
use strict; use warnings;
my @names = qw(Foo Bar Baz);
my $visitor = <STDIN>;
chomp $visitor;
if (grep { $visitor eq $_ } @names) {
print "Visitor $visitor is in the guest list\n";
} else {
print "Visitor $visitor is NOT in the guest list\n";
}
grep
그러나 해시에서 작동하는 똑같이 간단한 방법이 있는지 궁금합니다.해시의 각 항목을 반복하기 위해 루프를 작성할 필요가 없습니다..
다음은 제가 사용하고 있는 구조에 대한 몇 가지 샘플 데이터입니다. URI를 할당하기 전에 항목에 이미 해당 URI 값이 있는지 확인하고 싶습니다. 예를 들어,ww1.example.com
item 에 할당하고 싶지만 v2rbz1568
uri 값을 가진 다른 항목이 없는 경우에만 가능합니다 ww1.example.com
. Perl에서 이를 효율적으로 수행하려면 어떻게 해야 합니까?
{
"0y7vfr1234": {
"username": "[email protected]",
"password": "some-random-password123",
"uri": "ww1.example.com",
"index": 14
},
"v2rbz1568": {
"username": "[email protected]",
"password": "some-random-password125",
"uri": "ww3.example.com",
"index": 29
},
"0zjk1156": {
"username": "[email protected]",
"password": "some-random-password124",
"uri": "ww2.example.com",
"index": 38
}
}
저는 Linux에서 Perl 5 버전 30을 사용하고 있습니다.
답변1
최소한 두 가지 옵션이 있습니다:
- 귀하는 귀하의 질문에서 구상한 데이터 구조만을 가지고 있습니다. 그런 다음 일치하는 항목을 찾고 싶을 때마다 전체 "목록"을 반복해야 합니다. 그러나 루프를 작성할 필요는 없으며 다음
map
함수를 사용할 수 있습니다.
use strict; use warnings;
my %entries = (
'0y7vfr1234' => {
'username' => '[email protected]',
'password' => 'some-random-password123',
'uri' => 'ww1.example.com',
'index' => 14
},
'v2rbz1568' => {
'username' => '[email protected]',
'password' => 'some-random-password125',
'uri' => 'ww3.example.com',
'index' => 29
}
);
my $uri = <STDIN>;
chomp $uri;
if (grep { $uri eq $_ } map { $_->{'uri'} } values %entries) {
print "URI $uri is in the list\n";
} else {
print "URI $uri is NOT in the list\n";
}
- 빠른 조회가 가능하도록 해시에 별도의 인덱스를 관리할 수 있습니다. 인덱싱이란 URI를 항목의 실제 해시에 매핑하는 별도의 해시가 있음을 의미합니다.
use strict; use warnings;
my %entries = (
'0y7vfr1234' => {
'username' => '[email protected]',
'password' => 'some-random-password123',
'uri' => 'ww1.example.com',
'index' => 14
},
'v2rbz1568' => {
'username' => '[email protected]',
'password' => 'some-random-password125',
'uri' => 'ww3.example.com',
'index' => 29
}
);
my %index = map { $entries{"uri"} => $_ } keys %entries;
my $uri = <STDIN>;
chomp $uri;
my $item = $index{$uri};
if (defined($item)) {
print "URI $uri is in the list\n";
} else {
print "URI $uri is NOT in the list\n";
}
$item
해시가 존재하는지 확인하는 것 외에도 해시에 이미 존재하는 항목에 액세스하려는 경우 조회 결과를 직접 얻을 수 있기 때문에 편리합니다 .print "Index: ",$entries{$item}->{'index'},"\n";
두 번째 경우에는 "목록"에 URI가 추가/업데이트/제거될 때마다 인덱스를 수동으로 업데이트해야 합니다.
$entries{"v84x9v8b9"} = { uri => "ww49", ... };
$index{"ww49"} = "v84x9v8b9";